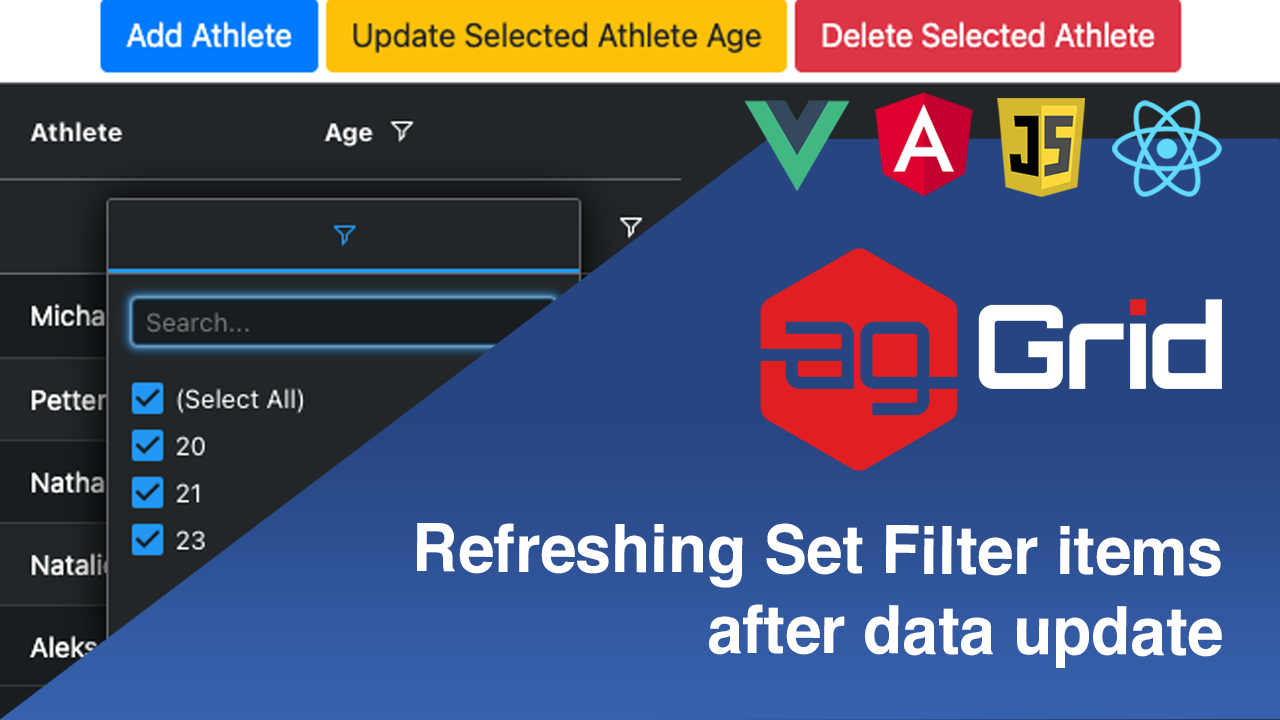
This post will show you how to refresh set filter items after a data update when you're using server-side row model in ag-Grid. Set filter items update automatically in response to data updates when you're using the client-side row model (use for datasets with fewer than 30,000 rows). However, when you're using the ag-Grid server-side row model, you need to implement this set filter update logic with user code.
In this blogpost we'll show you how to build exactly this user code. This way you can deliver a great filtering experience and make your application easy to use.
We will implement code for set filter refresh in response to adding, updating and deleting rows which match the filter condition.
Live example
Please see the live demo below, which is built in Vanilla JavaScript. In this sample, please edit the AGE column values or click the buttons above the grid to add/remove/update rows. Then, open the AGE column filter and see the filter values were updated in response to that data update.
You can also open the example implemented using other frameworks with the links below:
Let's see how to implement this for the different types of edits you can do.
Contents
- How does the Set Filter refresh?
- Adding a new row with a value matching the filter condition
- Updating the filtered column value of a row matching the filter condition
- Updating the filtered column value of a row matching the filter condition via Cell Editing
- Deleting a row which matches the filter condition
How does the Set Filter refresh?
There are a couple of ways to refresh the Set Filter items when there is a data update. These methods are detailed in our documentation.
Refresh set filter every time it is opened
The examples in this blog are refreshing the values every time the Set Filter is opened by adding the property refreshValuesOnOpen
to the filterParams
:
var columnDefs = [
// [...]
{
field: 'age',
filter: true,
filterParams: {
values: function (params) {
let asyncValues = fakeServer.getAges();
setTimeout(function () {
params.success(asyncValues);
}, 500);
},
refreshValuesOnOpen: true,
},
},
];
Refresh set filter values at a later point
If you want to avoid refreshing the Set Filters every time, you can refresh manually by getting the instance of the filter and calling the method refreshFilterValues()
as shown below:
let ageFilter = gridOptions.api.getFilterInstance('age');
ageFilter.refreshFilterValues();
See this in action in the sample below which has the Set Filter displayed at all times by enabling the Filter Tool Panel:
Adding a new row with a value matching the filter condition
When you add a new row which matches the filter condition, it needs to be displayed in the filtered grid.
Follow the steps below to see this in action:
- Open the Age column filter and select the value 20. The grid will then show all rows with age=20.
- Click on the Add Athlete button. The newly added row will be displayed in the filtered grid because it matches the filter condition (it also has an age of 20).
You can see this illustrated in the following GIF:
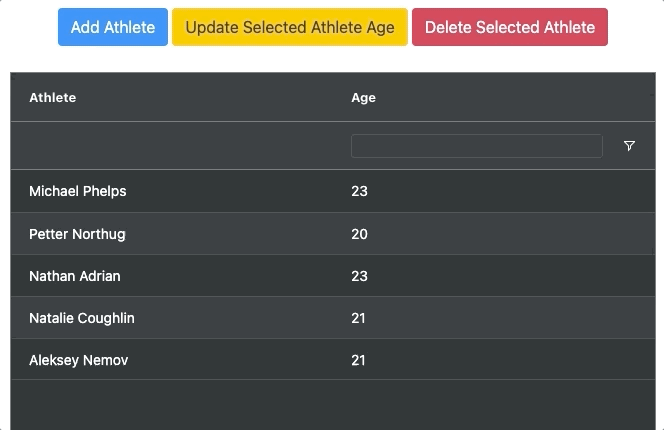
How it works
After adding the new row to the server-side data, we call purgeServerSideCache
to update this on the server and refresh the grid:
let athleteNames = ['Michael Phelps', 'Cindy Klassen', 'Nastia Liukin'];
function onBtnAddRow() {
// mimic server side adding row
const data = fakeServer.getData();
data.push({
id: data.length === 0 ? 0 : data[data.length - 1].id + 1,
athlete: athleteNames[data.length % athleteNames.length],
age: 20,
});
gridOptions.api.purgeServerSideCache();
}
Updating the filtered column value of a row matching the filter condition
Let's now see how ag-Grid and the set filter react to an update to one of the filtered rows via API.
Follow the steps below to see this in action:
- Open the Age column filter and check items 20 and 23. The grid will then show athletes with age of 20 OR 23
- Select an athlete with age=20 from the grid and click on the Update Selected Athlete Age button to update the age. The selected athlete will have its age incremented by 1 to now be 21.
This produces two effects:
- The selected athlete age has been updated to 21 and is subsequently removed from the view (as it no longer matches the filter condition - 20 OR 23)
- The Age set filter items are now 21 and 23, since the age value 20 is no longer present in the grid, and the value 21 is now present.
This is shown in the following GIF:
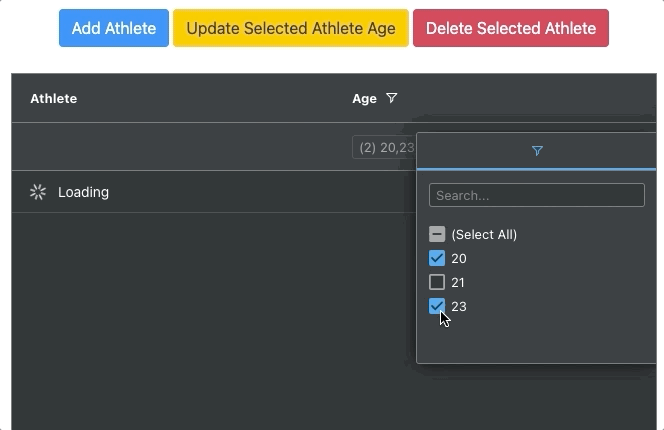
How it works
When the button is clicked, we find the selected rows in the grid based on their ID, increment the Age value, tell the server to update them (via mock method updateServerRows) and re-filter the grid by calling the onFilterChanged
event. This is shown below:
function onBtnUpdateData() {
var idsToUpdate = gridOptions.api.getSelectedNodes().map(function (node) {
return node.data.id;
});
// we are updating all the data
let updatedRows = [];
gridOptions.api.forEachNode((node) => {
if (idsToUpdate.indexOf(node.data.id) >= 0) {
let updated = { ...node.data };
updated.age += 1;
node.setData(updated);
updatedRows.push(updated);
}
});
// mimic server side update
updateServerRows(updatedRows);
gridOptions.api.onFilterChanged();
}
Updating the filtered column of a row matching the filter condition via Cell Editing
Another way of updating cells is via cell editing by pressing the Enter key or double clicking on the selected cell. See this in action in the GIF below:
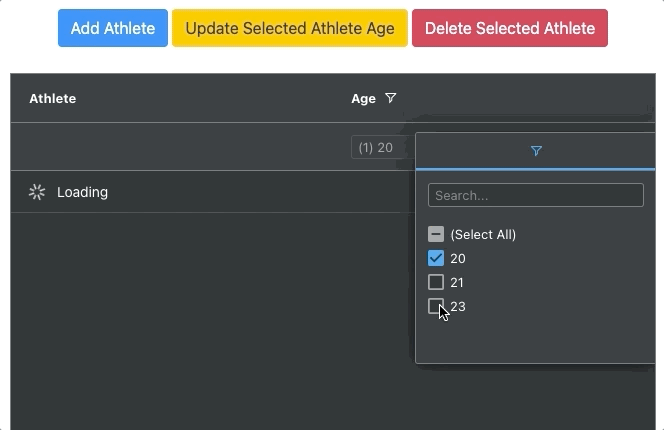
How it works
When a new value is set in the edited cell the grid event onCellValueChanged
is fired and we send the updated row data to the mock method updateServerRows to update the data from the mock server, followed by re-filtering the grid using the onFilterChanged
event.
var gridOptions = {
// ...,
onCellValueChanged: function (params) {
// trigger filtering on cell edits
let updatedRow = [{ ...params.data }];
updateServerRows(updatedRow);
params.api.onFilterChanged();
},
};
Deleting a row which matches the filter condition
When we delete the last row containing a value that appears in the set filter items, the ag-Grid set filter will remove this value from its items as it no longer appears in the grid.
Follow the steps below:
- Open the Age filter and select value 20. There is one row which passes the filter condition.
- Select that row and click the button Delete Selected Athlete. This will delete the row from the grid and since it was the only row in the grid with Age value of 20, the value 20 will be removed from the Set Filter items. Thi way the filter will only show 21 and 23 - the only Age values present in the grid)
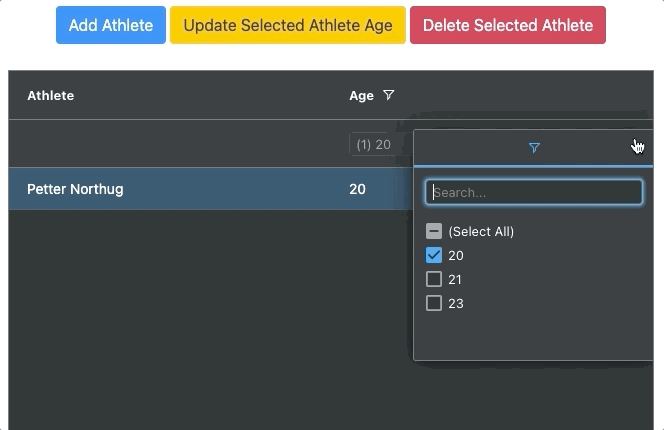
How it works
The row is deleted from the server-side data and the grid is refreshed by calling purgeServerSideCache
as implemented in the code below:
function onBtnDeleteSelectedRow() {
var selectedRows = gridOptions.api.getSelectedNodes();
const data = fakeServer.getData();
if (!selectedRows || selectedRows.length === 0) {
return;
}
var selectedRow = selectedRows[0];
var selectedRowId = selectedRow.data.id;
let index = data.findIndex((row) => row.id == selectedRowId);
if (index != -1) {
data.splice(index, 1);
}
gridOptions.api.purgeServerSideCache();
}
What's next?
We hope that you find this article useful whenever you're looking to work with set filters and the server-side row model in ag-Grid. Please use the samples above and modify them as you see fit.
If you would like to try out ag-Grid check out our getting started guides (JS / React / Angular / Vue)