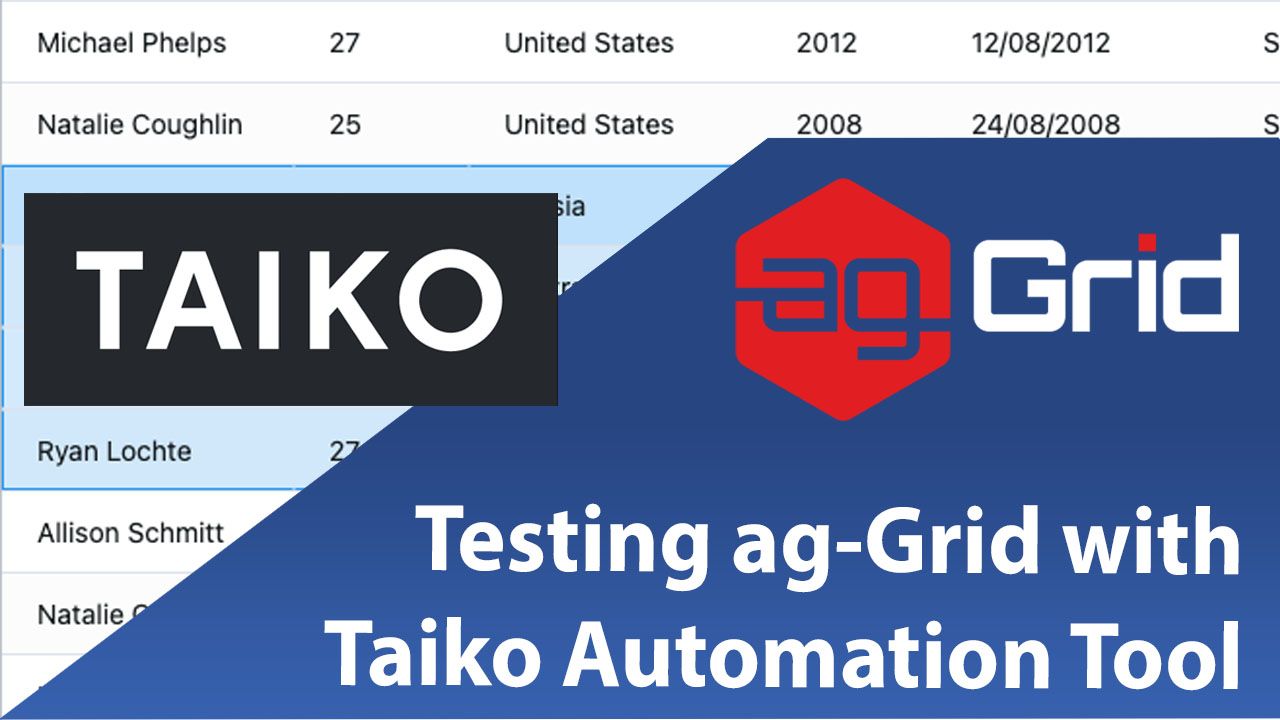
Taiko is a free and open source browser automation tool built by the team behind Gauge from ThoughtWorks. Taiko is a Node.js library with a clear and concise API to automate Chromium-based browsers (Chrome, Microsoft Edge, Opera) and Firefox. You integrate it with test runners like Gauge, Mocha, Jest to use it in a project.
In this post, we'll show you how to test ag-Grid with Taiko.
Testing ag-Grid with Taiko is illustrated for two test runners in these repos:
1. Testing ag-Grid with Taiko and Gauge
2. Testing ag-Grid with Taiko and Mocha
Contents
- Test Environment Setup
- Creating your first test script
- Sorting an ag-Grid column
- Filtering an ag-Grid column
- Grouping an ag-Grid column
- Identifying a row in ag-Grid
- Verifying text in a grid cell
- Selecting a value in a grid cell Combobox
Test Environment Setup
Prerequisites
- NodeJS
- A sample application using ag-Grid. To keep things simple we will be using the demo of ag-grid hosted on the ag-grid website.
Installation
Install taiko using npm by running the following command in a terminal:
$ npm install -g taiko
Creating the first test script
Start Taiko in REPL using the command below:
> taiko
Before executing a user flow we have to open the browser and visit the webpage to be tested. In this case we navigate to ag-grid’s sample website and click on the columns button to close the side filters.
See the commands to execute this listed below:
> taiko
> openBrowser()
> goto('https://www.ag-grid.com/example.php')
> click("Columns")
Now that we've covered the basics, let's record some more complex test scripts with ag-Grid.
Sorting an ag-Grid column
You can sort a column in ag-Grid by clicking its column header. Let's see how we can build a test script to do this automatically.
In the test sample you can sort the Language column by using the click API as follows:
> click("Language")
See this in action in the embedded video below:
Though the term “Language” appears more than once on the page, Taiko looks for the first visible occurrence before performing an operation. Since the filters on the side column are closed, `click(“Language”)` will click on the column with the heading “Language”.
To save all the steps to a script file and exit the REPL please run the commands below:
> .code sort-ag-grid.js
> .exit
Taiko exports the code as JavaScript, as shown below:
const { openBrowser, goto, click, closeBrowser } = require('taiko');
(async () => {
try {
await openBrowser();
await goto('https://www.ag-grid.com/example.php');
await click("Columns");
await click("Language");
}
catch (error) {
console.error(error);
}
finally {
await closeBrowser();
}
})();
You can run the script using the taiko command:
> taiko sort-ag-grid.js
Filtering an ag-Grid column
To filter a column in ag-grid, the manual steps are:
- Open the floating filter menu of the column Language. Since there are several filter menus, we identify it by specifying that it is below and to the right of the column Language.
- In the application all items are selected by default. We have to unselect the “Select All” checkbox before filtering on the required elements.
- Select the filter “English” in the column “Language”
- Select the filter “Greek” in the column “Language”
Let's now build a test script to do the same steps automatically. In the REPL we can start to automate the flow with the commands below:
> click(button({"aria-label": "Open Filter Menu"}), below("Language"), toRightOf("Language"))
> click(checkBox(toLeftOf(text("Select All",below("Language")))));
> click(checkBox(toLeftOf(text("English",below("Language")))));
> click(checkBox(toLeftOf(text("Greek",below("Language")))));
> .code filter-ag-grid.js
> .exit
See this in action in the video below:
This gives the following JavaScript code:
const { openBrowser, goto, click, button, below, toRightOf, text, toLeftOf, checkBox, closeBrowser } = require('taiko');
(async () => {
try {
await openBrowser();
await goto('https://www.ag-grid.com/example.php');
await click("Columns");
await click(button({"aria-label": "Open Filter Menu"}), below("Language"), toRightOf("Language"));
await click(checkBox(toLeftOf(text("Select All",below("Language")))));
await click(checkBox(toLeftOf(text("English",below("Language")))));
await click(checkBox(toLeftOf(text("Greek",below("Language")))));
}
catch (error) {
console.error(error);
}
finally {
await closeBrowser();
}
})();
Run the script using the command below:
> taiko filter-ag-grid.js
Grouping an ag-Grid column
You can group the grid's rows by a column manually by dragging the “Language” column and dropping it into the section that says ”Drag here to set row groups”.
Here's how to automate this column grouping flow in Taiko’s REPL:
> dragAndDrop("Language",into('Drag here to set row groups'))
> .code group-ag-grid.js
> .exit
The placeholder ‘Drag here to set row groups’ can be used to indicate an element. In this case, we will use it to identify the area where the dragged element needs to be dropped.
See this in action in the video below:
This script then produces the following JavaScript code:
const { openBrowser, goto, click, into, dragAndDrop, closeBrowser } = require('taiko');
(async () => {
try {
await openBrowser();
await goto('https://www.ag-grid.com/example.php');
await click("Columns");
await dragAndDrop("Language",into('Drag here to set row groups'));
}
catch (error) {
console.error(error);
}
finally {
await closeBrowser();
}
})();
You can run the generated script using the command below:
> taiko group-ag-grid.js
Identifying an ag-Grid row
In order to do any row-level operations, you need to identify a grid row first, and then a cell within a column in this row based on the col-id. Col-id is unique for the column and row-id is unique for a row. The within selector defines the boundary within which an element can be searched. This is used here to identify a cell under a given column heading in a given row.
For example the code below looks for the element under the column “Language“ within the 1st row.
$('[col-id="language"]'), within($('[row-id="0"]'))
Once the cell is identified, you can perform any operation of your choice, for example verifying its text or selecting a value from a combobox editor in the cell. This is demonstrated below.
Verifying text in a grid cell
Once you've identified a row using the step above, you may want to verify the text in a grid cell.
In the REPL we can start to automate this flow with the following code:
> $('[col-id="language"]', within($('[row-index="0"]'))).text()
> ‘English`
> .code assert-text-in-row.js
> .exit
See this in action in the video below:
This produces the following test code in JavaScript:
const { openBrowser, goto, click, $, within, closeBrowser } = require('taiko');
const assert = require(“assert”);
(async () => {
try {
await openBrowser();
await goto('https://www.ag-grid.com/example.php');
await click("Columns");
assert.equal(await ($('[col-id="language"]', within($('[row-index="0"]'))).text()),"English");
}
catch (error) {
console.error(error);
}
finally {
await closeBrowser();
}
})();
Note: `English` is the value of the text of the cell in row `0` under the column “Language”. We can assert on this by updating the above code snippet with the assertion statement.
Run the script using the command below:
> taiko assert-text-in-row.js
Selecting a value in a grid cell Combobox
Another action you can perform once you've identified a grid cell is selecting a specific option in a combobox editor in the cell. You can do that by following the steps below:
- Double click on the cell with the combobox for it to show the dropdown elements.
- Select the required value.
In the REPL we can automate the flow with the following code:
> doubleClick($('[col-id="language"]', within($('[row-index="1"]'))));
> click("Spanish");
> .code combobox-in-row.js
> .exit
See this in action in the video below:
The saved JavaScript file looks like this:
const { openBrowser, goto, click, $, within, closeBrowser, doubleClick } = require('taiko');
(async () => {
try {
await openBrowser();
await goto('https://www.ag-grid.com/example.php');
await click("Columns");
await doubleClick($('[col-id="language"]', within($('[row-index="1"]'))));
await click("Spanish");
}
catch (error) {
console.error(error);
}
finally {
await closeBrowser();
}
})();
Run the script using the command below:
> taiko combobox-in-row.js
What's next?
We hope this gives you a good introduction on how to use Taiko to generate tests against ag-Grid.
The samples illustrating how to use Taiko and Gauge to test ag-grid, Taiko and Mocha to test ag-grid are available on Github. These also demonstrate how to select a date. If you think we have missed any scenarios, please leave us a comment and we'll follow up with you.
If you would like to try out ag-Grid check out the getting started guides (JS / React / Angular / Vue)
Happy coding!