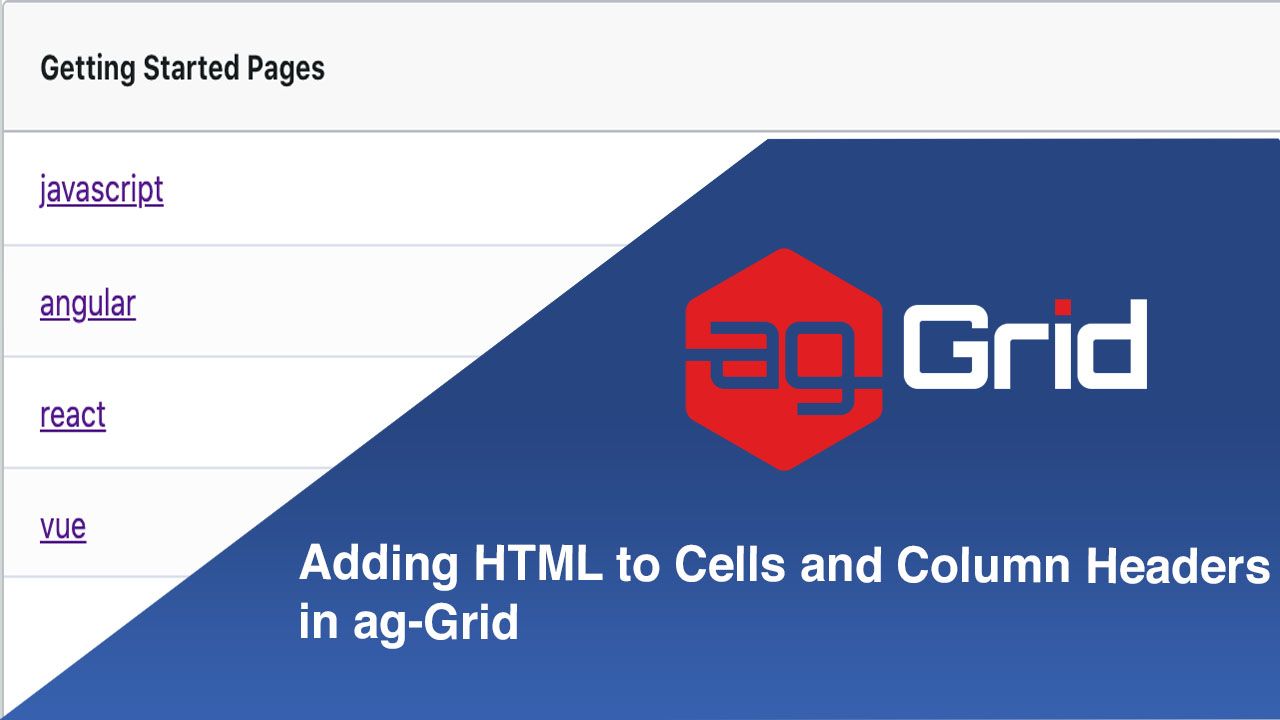
AG Grid allows you a lot of flexibility in the content you can render in cells and column headers. A common requirement is to display HTML code in a column of cells or as the column header itself. In this post, we'll show you how to render HTML code within both column headers and cells - you can see the final result of the former in the gif below and the latter in the image above. This blog is split into two sections:
HTML in Column Headers
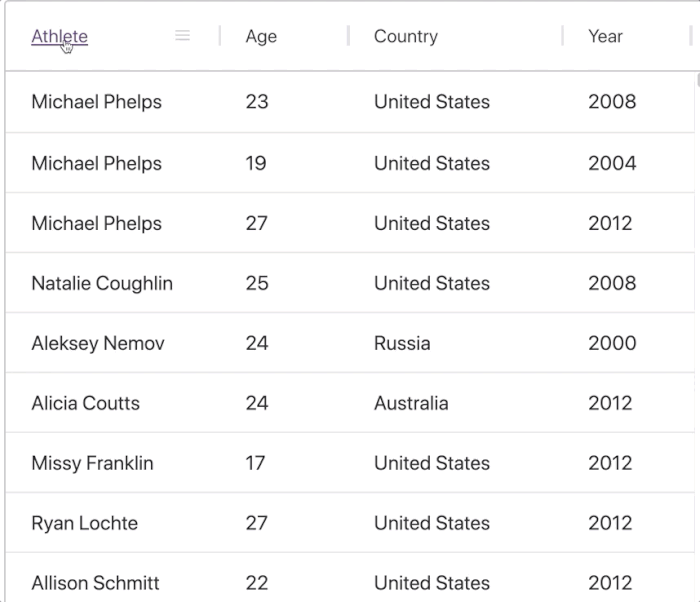
This behaviour is very easily achieved using a header template, which allows for simple UI customisations of the default header component.
//Athlete column definition
{
minWidth: 150,
field: 'athlete',
headerComponentParams: {
template:
'<div class="ag-cell-label-container" role="presentation">' +
' <span ref="eMenu" class="ag-header-icon ag-header-cell-menu-button"></span>' +
' <div ref="eLabel" class="ag-header-cell-label" role="presentation">' +
' <span ref="eSortOrder" class="ag-header-icon ag-sort-order" ></span>' +
' <span ref="eSortAsc" class="ag-header-icon ag-sort-ascending-icon" ></span>' +
' <span ref="eSortDesc" class="ag-header-icon ag-sort-descending-icon" ></span>' +
' <span ref="eSortNone" class="ag-header-icon ag-sort-none-icon" ></span>' +
' <a href="https://ag-grid.com" target="_blank"> <span ref="eText" class="ag-header-cell-text" role="columnheader"></span></a>' +
' <span ref="eFilter" class="ag-header-icon ag-filter-icon"></span>' +
' </div>' +
'</div>'
},
}
To use a header template we add it to the template property of the headerComponentParams
column property, in the column definitions of our chosen column (we chose the athlete column for this example). We've focused on customising the eText
element for this example (highlighted above), this is the text in the column header. To transform the value to a hyperlink we've simply wrapped it in an anchor tag.
That's all there is to it!
The example below was created using vanilla JS, please feel free to explore it! We've also prepared some examples using React, Angular and Vue.
Learn more about how to set up and customise column header templates here.
HTML in Cells
That covers one way of adding HTML to the grid but we still need to look at how to add hyperlinks to the grids cells.
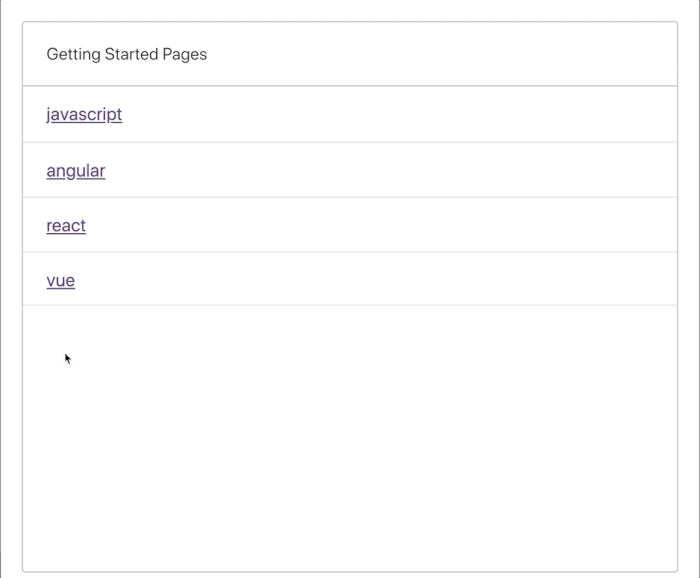
Once again, this behaviour is very easily implemented. This time, to achieve it we use a cell renderer. By default ag-grid will create the cell values using simple text, cell renderers allow us to add more complex HTML inside the cells.
As you'll see in our documentation, there are many ways to reference cell renderers in the column definitions of ag-grid, these include:
String
: The name of a cell renderer component.Class
: Direct reference to a cell renderer component.Function
: A function that returns either an HTML string or DOM element for display.
// 1 - String - The name of a cell renderer registered with the grid.
var colDef1 = {
cellRenderer: 'agGroupCellRenderer',
...
}
// 2 - Class - Provide your own cell renderer component directly without registering.
var colDef2 = {
cellRenderer: MyCustomCellRendererClass,
...
}
// 3 - Function - A function that returns an HTML string or DOM element for display
var colDef3 = function(params) {
// put the value in bold
return 'Value is <b>'+params.value+'</b>';
}
In this sample, we're going to use a function that returns a HTML string. For more information on the other two approaches, please see:
1. Referencing a cell renderer by name (docs)
2. Referencing a cell renderer by object reference (blog, docs)
cellRenderer: function(params) {
let keyData = params.data.key;
let newLink =
`<a href= https://ag-grid.com/${keyData}-getting-started
target="_blank">${keyData}</a>`;
return newLink;
}
In our example we're actually being a bit fancy and making different links for each cell by using the underlying data in the cells as can be seen in the code snippet above. The result is that the hyperlinks send users to our getting started pages for Vanilla JS, React, Vue and Angular depending on the cell clicked. This of course is optional, you don't have to use the cell's data to build the link.
The cellRenderer
property is added to the column definition of any column you wish to use it on along with the function returning the HTML and that is all there is to it!
Again, the example below was created using vanilla JS but we have also prepared examples using React, Angular, and Vue.
Learn more about creating custom cell renderers here and using our provided cell renderers here.
What's next?
I hope this article makes rendering HTML in ag-Grid more clear for you. Please check out our other blog posts and documentation for a great variety of features you can implement with ag-Grid.
If you would like to try out ag-Grid check out our getting started guides (JS / React / Angular / Vue)
Happy coding!