- Tempted to create your own Data Grid?
- What should you be doing instead?
- Pagination
- Filter, Search and Sort
- Responsive Table
- Infinite Scrolling
- Why should I use a library?
- What to look for before using a library?
- What separates good from great?
- Example
Tempted to create your own Data Grid?
So you've been assigned a task to create a web interface to display some data that you don't really care about.
You don't know how this data is gonna be useful to any one.
But you do know that the data is constantly changing and it is big, really big.
As a developer, you get excited thinking of all the different ways you can optimize and rock the user experience.
You write down all the requirements and then realize that there is so much on your plate.
In a real-world situation, business expectations and deadlines will dictate the amount of time you spend on a feature. A company might not prioritize your growth as a developer. You might want to write a feature yourself by scratch but you might not have the luxury to do that. This can be frustrating and can hinder your progress as a developer.
What should you be doing instead?
I would like every developer to create their own data table library at least once in their career so that they learn how complex seemingly simple features are. e.g. basic features like:
- Pagination
- Filter
- Sort
- Search
- Responsive Table
- Infinite scrolling
Lets go over each of them briefly
Pagination
Pagination is mandatory when the data you are working with is large. Specially on mobile devices, where the internet speed might not be fast enough, you will not want your users to wait more than 2 seconds. If it takes any longer than that, they are just gonna bounce.
Using the pagination technique, you can not only make the user experience better, but you can also give the user a sense of how big the data is and give them the ability to switch to whichever section of data they want.
There are two types of pagination:
- Server-side pagination
- Client-side pagination
Server-side pagination is faster, however, your backend team might not have the resources or time to setup the architecture required. Developing this form of pagination from scratch will teach you how to send API requests containing the correct parameters.
An example request would look something like this:
https://endpoint.com/getData?offset=0&count=10
Here, count is the total number of items requested from the API and offset is the starting point of the data.
Developing pagination requires you to increment or decrement the offset according to the current page the user is on.
So, given that the count is 10, if the user wants to go to page 4, your offset should naturally be 30.
Sending multiple requests to your backend will adversely affect the user experience. Hence, caching must be done for a page that has already been loaded previously. There are various caching strategies that can be applied here but it's out of the scope of this article.
Client-side pagination can be a viable option for some cases in which the total size of the bulk data is not big enough to slow down the response time from the backend. Or maybe your business requirements just do not care about the user experience that much. I have seen this happen in some in-house applications.
This type of pagination simply requires you to send a single API request to the backend and get all the data at once. You store this data in an array more often than not. Then, according to the current page, you increment the offset and show/hide the data by using CSS. In frameworks like Angular, you just have to make a public array like currentData and use it in the html like this:
<li *ngFor="let data of currentData; index as i;"> {{data.text}}</li>
The corresponding component.js file would look something like:
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
class ExampleComponent {
currentData = [
{
text: "test 1"
},
{
text: "test 2"
},
{
text: "test 3"
}
];
offset = 0;
itemPerPage = 10;
}
For simplicity, I did not add the API request here.
Using *ngIf and and changing the offset variable, you can show/hide the data to your user and create a more organized experience for your user.
Filter, Search and Sort
Filter and sort features are almost always necessary in any table format data representation.
For sort and filter, you yet again have the same two options.
- Server-side Filter/Sort
- Client-side Filter/Sort
Server-side Filter/Sort is done by sending an API request to your backend. Your backend developer will filter/sort the data using database queries according to your needs (which are defined in the query string of your API request) and send you back the results. You can then just show them to the user. Also, caching the results might be useful again.
Client-side Filter/Sort is done on the data that has been requested from the backend. This data can be requested in chunks or in bulk depending on the load strategy (bulk/pagination/lnfinite scrolling) you are using.
As a developer, sorting might get tricky here if the data you will sort has dynamic types (strings/numbers/emojis). If you are using the Array.sort() method, you can use the callback function that this function takes in and customize the way it sorts your data. As far as filter/search is concerned, there are many options available. If you are using Angular, you will have to create a custom Pipe and modify it according to your use case. A good place to start is this Stackoverflow answer.
Responsive Table
Your data table has to be responsive if you are looking to reach the other half of your audience. As a business these days, most of your audience is using your application from their mobile devices. A responsive application is no longer a luxury item, rather it's a no-brainer.
Making a data-table responsive can be tricky if your column count is high. You will need to employ a few strategies to show the necessary data to your user while not robbing them of a pleasant experience in your application. These strategies are decided by you and your managers together. They usually listen to the developers more in this case, as some methods are quicker than others.
One of the ways we made our data table (containing 8 columns) fit onto a mobile screen was to just show 3 columns on the first load. The rows are clickable and initiates an animation similar to a drop-down menu. This dropdown style row will show the data of the remaining 5 columns in a more readable format. The choice of which data to show on first load was made by our managers. This overall strategy was really appreciated by our users after the release.
Making a table responsive from scratch will require quite a bit of effort. You will need to consider mobile devices, tablets and landscape/portrait orientations. So be careful and test it as much as you can :)
Infinite Scrolling
Sometimes, pagination is not the right way to go. You might want to show a list of games or movies. The list is long and you want the users to see the data in the order you have decided. You do not want your users to jump to a specific portion of the data, nor do you want them to know how much data there is. Instead of pagination you can implement Infinite scrolling.
Facebook, Twitter and Instagram use this extensively in their feeds where you scroll down to see content. All of that content is not loaded on the first load. If it was, you would probably have to wait a couple of minutes before you see anything on your feed.
Infinite scrolling is done as you scroll down and just before you reach the end of the page, you ask the backend API for more data. You show a loading indicator to signal the user that more data is on its way. If there is no data left to fetch, you just remove the loading indicator and don't send API requests any further.
Developing this feature from scratch will teach you a lot about how the onScroll() method on your browser works. Once you work out the position at which a new request should be sent, you will wait for the response to come back to the client. In this period, you will show the loading indicator while not blocking the UI thread. Your Async programming skills will be used here. The user should freely be able to scroll and interact with your application in this period. When the response comes, you need to add the data into your existing data (that the user is already seeing). If no data comes you need to add a flag to the page not to send more API requests.
Caching can be done here too and is highly recommended.
After you have done this exercise you will have a sense of how a library actually works behind the scenes and what it can/can't do. Developing these features will make you solve a variety of problems that you will face daily as a professional frontend developer. This will guide you into choosing the correct library for your use case.
Why should I use a library?
Now why should you use a library in the first place?
As I mentioned in the beginning, in a real-world situation, you will have to meet deadlines and produce results, specially if you are working in an agile setting.
Secondly, while creating your own library, you will realize the common pitfalls and edge-cases you might encounter in an actual application.
Data table libraries help you in speeding up your development. It helps you in focusing on the task at hand rather than struggling with the nuts and bolts. The library takes care of those behind the scenes.
What to look for before using a library?
A good developer will do their research when choosing a library. Common things to look for are
- Documentation
- Features the library provides
- Github stars
- Npm downloads
- Open issues on github
- Contributors/Pull requests
- Number of commits
- Frequency of commits
- Date of last commit
- Questions asked on stackoverflow
- Test coverage and tests passing
- Size of the library
- Support
Most newbie developers search for a library and then download the first one that has the feature they want.
What separates good from great?
As you use a third party library in your project, you are the one who is responsible if something goes wrong or if something is required that is not in the library, You should be able to edit and shape the features in the library to suit your specific needs. A good developer knows this and weighs the risks accordingly.
Example
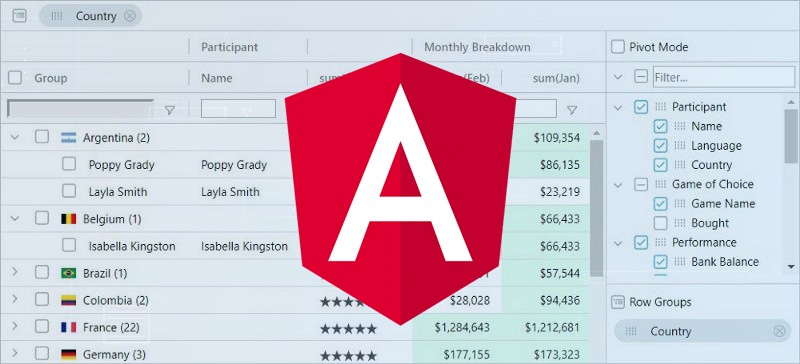
One such example of a data table solution is ag-Grid. Packed with features and performance users expect, you can't go wrong with ag-Grid, that's the data grid that I use and is fast becoming industry standard.
Learn about AG Grid Angular Support here, we have an Angular Quick Start Guide and all our examples have Angular versions.