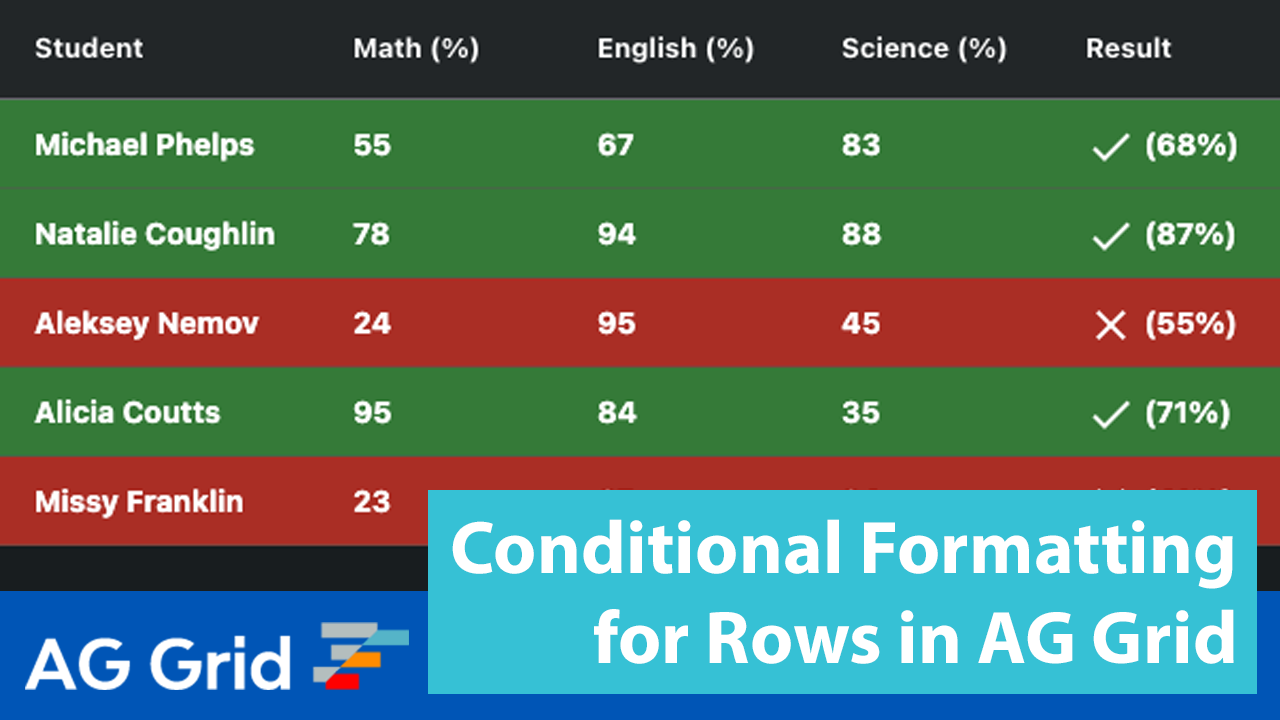
This post shows how to conditionally format rows in AG Grid. This feature is especially useful when your users need to quickly identify specific records for further processing. We'll look at how to implement the most frequent conditional formatting scenarios.
Note: We have a separate blogpost about conditional formatting for cells in AG Grid - see it here.
Contents
- Row Conditional Formatting in Action
- JavaScript Example of Conditional Row Formatting
- Setting row style based on cell value
- Changing row style after cell value update
Row Conditional Formatting in Action
We have illustrated these scenarios in a live sample in JavaScript. Please see it in action below:
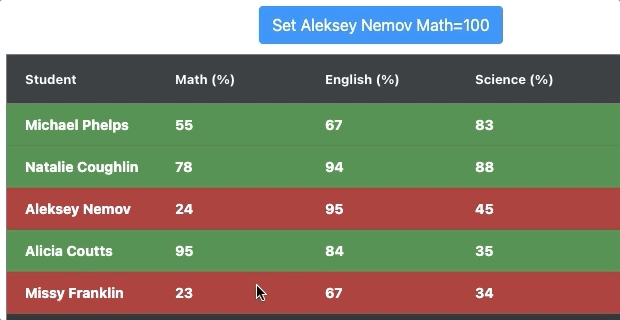
JavaScript Example of Conditional Row Formatting
See the live sample in JavaScript below.
Since this is a working example, try changing the values of the Math, English and Science results. The row colour is based on the average of all three test results. We also added some validation to check that the values are between 0 and 100.
Click the link in the bottom right corner of the sample below to open it in StackBlitz. You’ll see the code we've used to configure the grid so that only some cells are editable, how we aggregate the results and how we apply the conditional formatting. You can then change the code to set any additional row formatting rules you'd like to add.
Let's now look at the most common row conditional formatting scenarios and how to implement them in AG Grid.
Setting row style based on cell value
This is the most common conditional formatting scenario - you apply a style (for example set the background colour) to a row based on a cell value.
There are three ways of styling rows - by applying rowStyle
, rowClass
or rowClassRules
, documented here. While all these approaches will work, we highly recommend using rowClassRules
as it requires the least amount of code and the grid manages adding and removing the applied styles for you, as documented here.
So, let's style a row based on its result column value as follows:
1) If result value is greater than or equal to 60 the row will have a green background colour
2) If result value is less than 60 the row will have a red background colour
We implement this styling logic by setting rowClassRules
in gridOptions as shown below:
// index.js
var gridOptions = {
// [...]
rowClassRules: {
"row-fail": params => params.api.getValue("result", params.node) < 60,
"row-pass": params => params.api.getValue("result", params.node) >= 60
},
};
In the code above, row-fail
and row-pass
are the CSS classes to be applied to rows based on those conditions. These CSS classes currently only set the background color and set the font to bold, but you can easily add any other formatting setting you need to be applied. These CSS classes are defined as shown below:
/* style.css */
.row-fail {
font-weight: bold;
background-color: #aa2e25 !important;
}
.row-pass {
font-weight: bold;
background-color: #357a38 !important;
}
Et voila! Simple as that, all the rows in our Grid are now colored based on their result cell value as shown below:
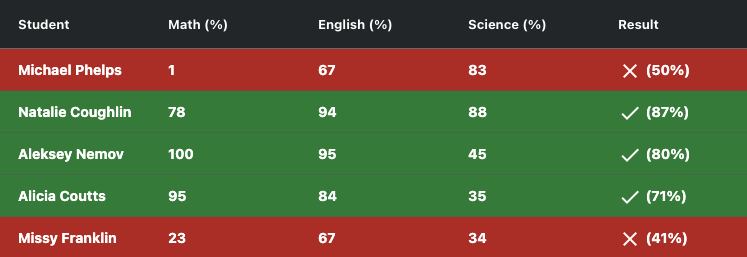
Changing row style after cell value update
Now that we've styled a row based on a static value, let's take a look at updating styles depending on updates in the result cell value.
When you refresh a row with new data, or a cell is updated due to editing, the rowClassRules
are refreshed automatically and the correct CSS classes are applied according to the styling conditions set. This means you don't need to write any extra code to support style updates when grid values are updated.
See this in action in the GIF below where we are performing two actions:
- Update the 'Michael Phelps' row with Math=1 to reduce the overall result value, causing the row background to change to red
- Update the 'Aleksey Nemov' row with Math=100 via a button click to increase the overall result, causing the row background to change to green
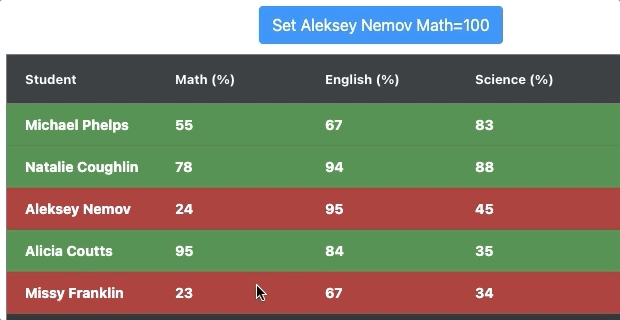
What's next?
We hope that you find this article useful to implement conditional formatting for rows in AG Grid. Please use the samples in the blogpost and modify them as you see fit.
For more information on how to style rows please see the row styling documentation, as well as the rowClassRules documentation section with a live example using rowClassRules with data updates.
If you would like to try out ag-Grid check out our getting started guides (JS / React / Angular / Vue)
Happy coding!