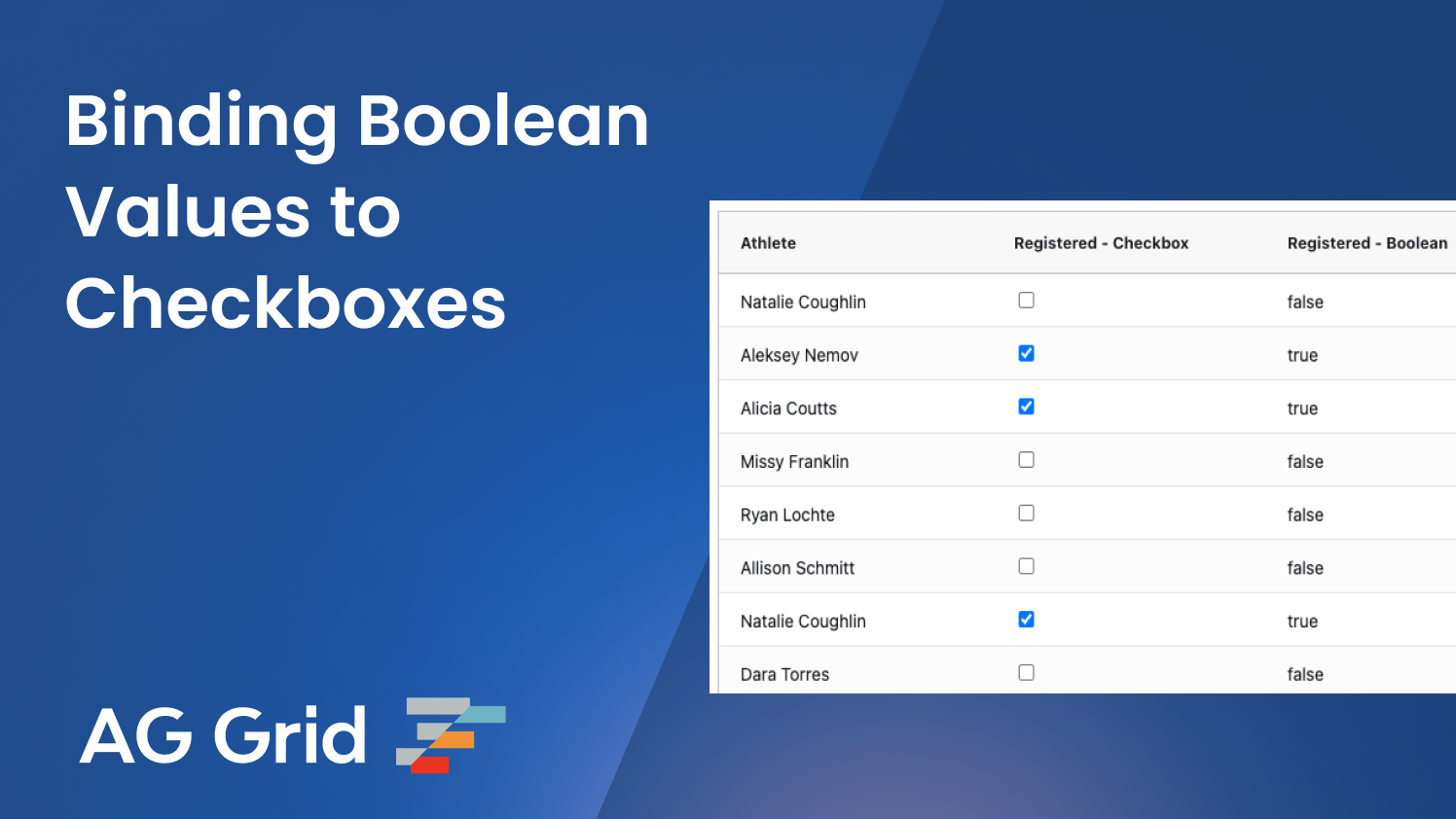
Binding boolean values to checkboxes in AG Grid is easy. You can display boolean values with a checkbox using one of the AG Grid provided cell renderers.
Cell Renderers are used when you want AG Grid to display more than just text within a cell. You can use cell renderers to add checkboxes, buttons, or images within your grid cells.
The AG Grid documentation pages show how to use the provided Checkbox Cell Renderer in all the different frameworks:
- React Checkbox Cell Renderer
- Angular Checkbox Cell Renderer
- Vue Checkbox Cell Renderer
- Vanilla JavaScript Checkbox Cell Renderer
What's next?
I hope you find this article useful when binding boolean values to checkboxes in AG Grid. Please check out our documentation for a great variety of scenarios you can implement with AG Grid.
If you would like to try out AG Grid check out our getting started guides (JS / React / Angular / Vue)
Happy coding!